- プロンプト実行例
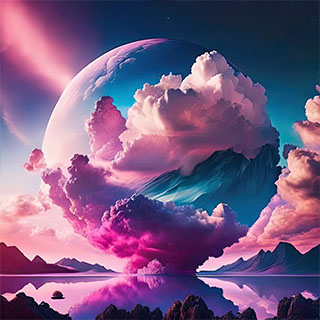
スクリプトを複数ファイルにまとめます。
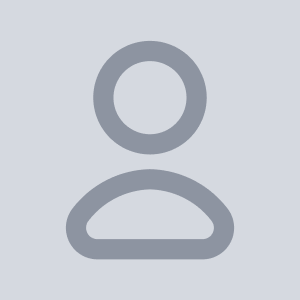
var charCount = inputText.length;
var charCountNoSpace = inputText.replace(/\s/g, '').length;
var wordCount = inputText.trim().split(/\s+/).length;
var lineBreakCount = (inputText.match(/\n/g) || []).length;
var alphabetCount = inputText.replace(/[^A-Za-z]/g, '').length;
var numberCount = inputText.replace(/[^0-9]/g, '').length;
var hiraganaCount = inputText.replace(/[^ぁ-んァ-ン]/g, '').length;
var katakanaCount = inputText.replace(/[^ァ-ン]/g, '').length;
var punctuationCount = inputText.replace(/[^。、.,-]/g, '').length;
var symbolCount = inputText.replace(/[^!-]/g, '').length;
var kanjiCount = inputText.replace(/[^一-龠々]/g, '').length;
var urlCount = inputText.split('http').length - 1;
var utf8ByteCount = new Blob([inputText], {type: 'plain/text'}).size;
var shiftJISByteCount = unescape(encodeURIComponent(inputText)).length;
var jisByteCount = inputText.replace(/[^ -。-゚]/g, '').length;
var eucJPByteCount = encodeURIComponent(inputText).replace(/%[A-F\d]{2}/g, 'U').length;
var charPerPage = parseFloat(document.getElementById("charPerPage").value); var includeHalfWidth = document.getElementById("includeHalfWidth").checked;
var pageCount = Math.ceil(charCount / charPerPage); if (includeHalfWidth) { charCount += inputText.replace(/[^\x01-\x7E]/g, '').length * -0.5; }
document.getElementById("charCount").textContent = charCount; document.getElementById("charCountNoSpace").textContent = charCountNoSpace; document.getElementById("wordCount").textContent = wordCount; document.getElementById("lineBreakCount").textContent = lineBreakCount; document.getElementById("alphabetCount").textContent = alphabetCount; document.getElementById("numberCount").textContent = numberCount; document.getElementById("hiraganaCount").textContent = hiraganaCount; document.getElementById("katakanaCount").textContent = katakanaCount; document.getElementById("punctuationCount").textContent = punctuationCount; document.getElementById("symbolCount").textContent = symbolCount; document.getElementById("kanjiCount").textContent = kanjiCount; document.getElementById("urlCount").textContent = urlCount; document.getElementById("utf8ByteCount").textContent = utf8ByteCount; document.getElementById("shiftJISByteCount").textContent = shiftJISByteCount; document.getElementById("jisByteCount").textContent = jisByteCount; document.getElementById("eucJPByteCount").textContent = eucJPByteCount; document.getElementById("pageCount").textContent = pageCount; } </script>
</head> <body> <textarea id="input" rows="10" cols="50" placeholder="ここにテキストを入力してください"></textarea> <br> <button onclick="countCharacters()">文字数をカウント</button> <br> <label for="charPerPage">1ページあたりの文字数: </label> <input type="number" id="charPerPage" value="400"> <label><input type="checkbox" id="includeHalfWidth">半角文字を0.5文字としてカウントする</label> <br> <p>文字数(スペース込み): <span id="charCount"></span></p> <p>文字数(スペース無視): <span id="charCountNoSpace"></span></p> <p>単語数: <span id="wordCount"></span></p> <p>改行コード数: <span id="lineBreakCount"></span></p> <p>英字数: <span id="alphabetCount"></span></p> <p>数字数: <span id="numberCount"></span></p> <p>ひらがな数: <span id="hiraganaCount"></span></p> <p>カタカナ数: <span id="katakanaCount"></span></p> <p>句読点数: <span id="punctuationCount"></span></p> <p>記号数: <span id="symbolCount"></span></p> <p>漢字数: <span id="kanjiCount"></span></p> <p>URL数: <span id="urlCount"></span></p> <p>UTF-8バイト数: <span id="utf8ByteCount"></span></p> <p>Shift_JISバイト数: <span id="shiftJISByteCount"></span></p> <p>JISバイト数: <span id="jisByteCount"></span></p> <p>EUC_JPバイト数: <span id="eucJPByteCount"></span></p> <p>原稿用紙何枚: <span id="pageCount"></span></p> </body> </html>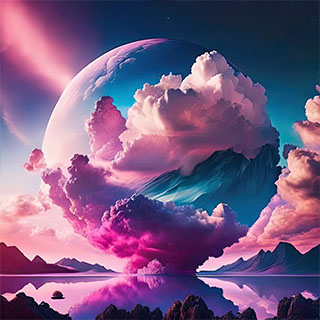
html1<!-- index.html --> 2<!DOCTYPE html> 3<html lang="ja"> 4<head> 5 <meta charset="UTF-8"> 6 <title>文字数カウントツール</title> 7 <link rel="stylesheet" type="text/css" href="styles.css"> 8</head> 9<body> 10 <textarea id="input" rows="10" cols="50" placeholder="ここにテキストを入力してください"></textarea> 11 <br> 12 <button onclick="countCharacters()">文字数をカウント</button> 13 <br> 14 <label for="charPerPage">1ページあたりの文字数: </label> 15 <input type="number" id="charPerPage" value="400"> 16 <label><input type="checkbox" id="includeHalfWidth">半角文字を0.5文字としてカウントする</label> 17 <br> 18 <p>文字数(スペース込み): <span id="charCount"></span></p> 19 <p>文字数(スペース無視): <span id="charCountNoSpace"></span></p> 20 <p>単語数: <span id="wordCount"></span></p> 21 <p>改行コード数: <span id="lineBreakCount"></span></p> 22 <p>英字数: <span id="alphabetCount"></span></p> 23 <p>数字数: <span id="numberCount"></span></p> 24 <p>ひらがな数: <span id="hiraganaCount"></span></p> 25 <p>カタカナ数: <span id="katakanaCount"></span></p> 26 <p>句読点数: <span id="punctuationCount"></span></p> 27 <p>記号数: <span id="symbolCount"></span></p> 28 <p>漢字数: <span id="kanjiCount"></span></p> 29 <p>URL数: <span id="urlCount"></span></p> 30 <p>UTF-8バイト数: <span id="utf8ByteCount"></span></p> 31 <p>Shift_JISバイト数: <span id="shiftJISByteCount"></span></p> 32 <p>JISバイト数: <span id="jisByteCount"></span></p> 33 <p>EUC_JPバイト数: <span id="eucJPByteCount"></span></p> 34 <p>原稿用紙何枚: <span id="pageCount"></span></p> 35 <script src="scripts.js"></script> 36</body> 37</html>
css1/* styles.css */ 2/* Add your styles here */
JavaScript1// scripts.js 2function countCharacters() { 3 var inputText = document.getElementById("input").value; 4 5 var charCount = inputText.length; 6 var charCountNoSpace = inputText.replace(/\s/g, '').length; 7 var wordCount = inputText.trim().split(/\s+/).length; 8 var lineBreakCount = (inputText.match(/\n/g) || []).length; 9 var alphabetCount = inputText.replace(/[^A-Za-z]/g, '').length; 10 var numberCount = inputText.replace(/[^0-9]/g, '').length; 11 var hiraganaCount = inputText.replace(/[^ぁ-んァ-ン]/g, '').length; 12 var katakanaCount = inputText.replace(/[^ァ-ン]/g, '').length; 13 var punctuationCount = inputText.replace(/[^。、.,\-]/g, '').length; 14 var symbolCount = inputText.replace(/[^!-~]/g, '').length; 15 var kanjiCount = inputText.replace(/[^一-龠々]/g, '').length; 16 var urlCount = inputText.split('http').length - 1; 17 var utf8ByteCount = new Blob([inputText], {type: 'plain/text'}).size; 18 var shiftJISByteCount = unescape(encodeURIComponent(inputText)).length; 19 var jisByteCount = inputText.replace(/[^ -~。-゚]/g, '').length; 20 var eucJPByteCount = encodeURIComponent(inputText).replace(/%[A-F\d]{2}/g, 'U').length; 21 22 var charPerPage = parseFloat(document.getElementById("charPerPage").value); 23 var includeHalfWidth = document.getElementById("includeHalfWidth").checked; 24 25 var pageCount = Math.ceil(charCount / charPerPage); 26 if (includeHalfWidth) { 27 charCount += inputText.replace(/[^\x01-\x7E]/g, '').length * -0.5; 28 } 29 30 document.getElementById("charCount").textContent = charCount; 31 document.getElementById("charCountNoSpace").textContent = charCountNoSpace; 32 document.getElementById("wordCount").textContent = wordCount; 33 document.getElementById("lineBreakCount").textContent = lineBreakCount; 34 document.getElementById("alphabetCount").textContent = alphabetCount; 35 document.getElementById("numberCount").textContent = numberCount; 36 document.getElementById("hiraganaCount").textContent = hiraganaCount; 37 document.getElementById("katakanaCount").textContent = katakanaCount; 38 document.getElementById("punctuationCount").textContent = punctuationCount; 39 document.getElementById("symbolCount").textContent = symbolCount; 40 document.getElementById("kanjiCount").textContent = kanjiCount; 41 document.getElementById("urlCount").textContent = urlCount; 42 document.getElementById("utf8ByteCount").textContent = utf8ByteCount; 43 document.getElementById("shiftJISByteCount").textContent = shiftJISByteCount; 44 document.getElementById("jisByteCount").textContent = jisByteCount; 45 document.getElementById("eucJPByteCount").textContent = eucJPByteCount; 46 document.getElementById("pageCount").textContent = pageCount; 47}
コメント 0
他のプロンプトもチェック
文案作成
推奨モデル - GPT 4oカンニングペーパーのように、AIが文章を自動で考えてくれます!!あとは少し修正するだけ!!2029366画像生成
キャラクター作成
▼最新作はこちら▼ https://oshiete.ai/item_lists/73557973863457792 あなたの住んでいる都道府県を入力してみてください。AIが考える各都道府県のイメージが出力されます。232100739