スクリプト・複数ファイルをひとつのファイルに
HTML・CSS・JavaScript等が複数ファイルに分かれたものをひとつのファイルにまとめられます。 使用用途としては、ノーコードサイト等ではCSSやJavaScriptを単体では置けないことがあります。故にCSSとJavaScriptも置きたい!って時 一応 HTMLを含めてひとつのファイルにまとめると設置できるようになります
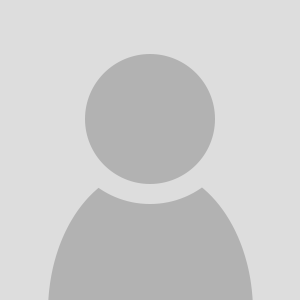
投稿日時:
- プロンプト実行例
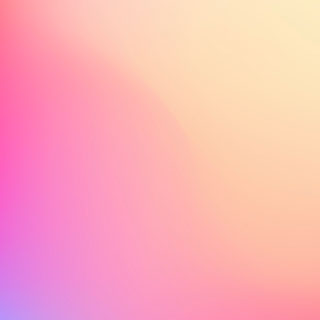
スクリプトをひとつのファイルにまとめます。
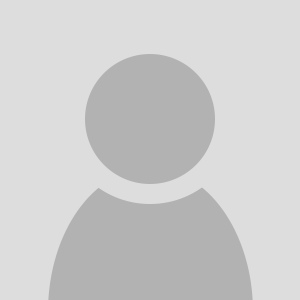
</body> </html>1 <table id="board"></table> 2 </div> 3 <div id="counter" class="hide"> 4 <div id="numBlack"></div> 5 <h2></h2> 6 <div id="numWhite"></div> 7 </div> 8</main> 9 10<script src="main.js"></script>
"use strict";
const BLACK = 1, WHITE = -1; let data = []; let turn = true; const board = document.getElementById("board"); const h2 = document.querySelector("h2"); const counter = document.getElementById("counter"); const modal = document.getElementById("modal"); document.querySelectorAll(".select").forEach((value) => { value.addEventListener("click", start); }); let cells = 8; // マスの数
// スタート画面でマスの数が選択された時の処理 function start(e) { cells = Number(e.target.id); board.innerHTML = ""; init(); modal.classList.add("hide"); }
// 初期化 function init() { for (let i = 0; i < cells; i++) { const tr = document.createElement("tr"); data[i] = Array(cells).fill(0); for (let j = 0; j < cells; j++) { const td = document.createElement("td"); const disk = document.createElement("div"); tr.appendChild(td); td.appendChild(disk); td.className = "cell"; td.onclick = clicked; } board.appendChild(tr); } // マスの数によって石の初期配置を変える switch (cells) { case 4: board.classList.add("cell-4"); putDisc(1, 1, WHITE); putDisc(2, 2, WHITE); putDisc(1, 2, BLACK); putDisc(2, 1, BLACK); break; case 6: board.classList.add("cell-6"); putDisc(2, 2, WHITE); putDisc(3, 3, WHITE); putDisc(2, 3, BLACK); putDisc(3, 2, BLACK); break; case 8: putDisc(3, 3, WHITE); putDisc(4, 4, WHITE); putDisc(3, 4, BLACK); putDisc(4, 3, BLACK); } showTurn(); }
init();
// 石を描画 function putDisc(x, y, color) { board.rows[y].cells[x].firstChild.className = color === BLACK ? "black" : "white"; board.rows[y].cells[x].animate( { opacity: [0.4, 1] }, { duration: 700, fill: "forwards" } ); data[y][x] = color; }
// 手番などの表示 function showTurn() { h2.textContent = turn ? "黒の番です" : "白の番です"; let numWhite = 0, numBlack = 0, numEmpty = 0; for (let x = 0; x < cells; x++) { for (let y = 0; y < cells; y++) { if (data[x][y] === WHITE) { numWhite++; } if (data[x][y] === BLACK) { numBlack++; } if (data[x][y] === 0) { numEmpty++; } } } document.getElementById("numBlack").textContent = numBlack; document.getElementById("numWhite").textContent = numWhite;
let blacDisk = checkReverse(BLACK); let whiteDisk = checkReverse(WHITE);
if (numWhite + numBlack === cells * cells || (!blacDisk && !whiteDisk)) { if (numBlack > numWhite) { document.getElementById("numBlack").textContent = numBlack + numEmpty; h2.textContent = "黒の勝ち!!"; restartBtn(); showAnime(); } else if (numBlack < numWhite) { document.getElementById("numWhite").textContent = numWhite + numEmpty; h2.textContent = "白の勝ち!!"; restartBtn(); showAnime(); } else { h2.textContent = "引き分け"; restartBtn(); showAnime(); } return; } if (!blacDisk && turn) { h2.textContent = "黒スキップ"; showAnime(); turn = !turn; setTimeout(showTurn, 2000); return; } if (!whiteDisk && !turn) { h2.textContent = "白スキップ"; showAnime(); turn = !turn; setTimeout(showTurn, 2000); return; } }
// マスがクリックされた時の処理 function clicked() { const color = turn ? BLACK : WHITE; const y = this.parentNode.rowIndex; const x = this.cellIndex; // マスに置けるかチェック if (data[y][x] !== 0) { return; } const result = checkPut(x, y, color); if (result.length > 0) { result.forEach((value) => { putDisc(value[0], value[1], color); }); turn = !turn; } showTurn(); }
// 置いたマスの周囲8方向をチェック function checkPut(x, y, color) { let dx, dy; const opponentColor = color == BLACK ? WHITE : BLACK; let tmpReverseDisk = []; let reverseDisk = []; // 周囲8方向を調べる配列 const direction = [ [-1, 0], // 左 [-1, 1], // 左下 [0, 1], // 下 [1, 1], // 右下 [1, 0], // 右 [1, -1], // 右上 [0, -1], // 上 [-1, -1], // 左上 ];
// すでに置いてある if (data[y][x] === BLACK || data[y][x] === WHITE) { return []; } // 置いた石の周りに違う色の石があるかチェック for (let i = 0; i < direction.length; i++) { dx = direction[i][0] + x; dy = direction[i][1] + y; if ( dx >= 0 && dy >= 0 && dx <= cells - 1 && dy <= cells - 1 && opponentColor === data[dy][dx] ) { tmpReverseDisk.push([x, y]); tmpReverseDisk.push([dx, dy]); // 裏返せるかチェック while (true) { dx += direction[i][0]; dy += direction[i][1]; if ( dx < 0 || dy < 0 || dx > cells - 1 || dy > cells - 1 || data[dy][dx] === 0 ) { tmpReverseDisk = []; break; } if (opponentColor === data[dy][dx]) { tmpReverseDisk.push([dx, dy]); } if (color === data[dy][dx]) { reverseDisk = reverseDisk.concat(tmpReverseDisk); tmpReverseDisk = []; break; } } } } return reverseDisk; }
// 裏返せる場所があるか確認 function checkReverse(color) { for (let x = 0; x < cells; x++) { for (let y = 0; y < cells; y++) { const result = checkPut(x, y, color); console.log(result); if (result.length > 0) { return true; } } } return false; }
// ゲーム終了画面 function restartBtn() { const restartBtn = document.getElementById("restartBtn"); restartBtn.classList.remove("hide"); restartBtn.animate( { opacity: [1, 0.5, 1] }, { delay: 2000, duration: 3000, iterations: "Infinity" } );
restartBtn.addEventListener("click", () => { document.location.reload(); }); } function showAnime() { h2.animate({ opacity: [0, 1] }, { duration: 500, iterations: 4 }); }
body { text-align: center; } main { display: inline-block; position: relative; }
/* start */ #modal { position: absolute; top: 0; left: 0; width: 100%; height: 100%; background: rgba(31, 19, 19, 0.8); color: white; }
#menu { display: flex; justify-content: space-evenly; margin-top: 220px; } h3, p { color: white; } #menu p { width: 100px; font-size: 24px; padding: 3px; margin: 15px; border: solid 1px white; cursor: pointer; background: black; color: white; }
/* game */ #board { margin: 0 auto; background: #555555; } td.cell { background: rgb(128, 216, 154); width: 46px; height: 46px; margin: 2px; } .cell div { width: 35px; height: 35px; border-radius: 50%; margin: 0 auto; } .cell-6 > tr > td { width: 63px; height: 63px; } .cell-6 > tr > td > div { width: 50px; height: 50px; } .cell-4 > tr > td { width: 92px; height: 92px; } .cell-4 > tr > td > div { width: 70px; height: 70px; } .black { background: black; } .white { background: white; } h2 { text-align: center; margin: 5px 0; } #counter { margin: 0 auto; width: 350px; display: flex; line-height: 50px; font-weight: bold; justify-content: space-between; } #numBlack { font-size: 36px; margin: 5px; width: 100px; height: 50px; background: black; color: white; border-radius: 5px; } #numWhite { font-size: 36px; margin: 5px; width: 100px; height: 50px; border: solid 1px black; border-radius: 5px; }
/* end */ #restartBtn { z-index: 1; opacity: 0; position: absolute; width: 100%; height: 50px; line-height: 50px; font-weight: bold; cursor: pointer; top: 50%; left: 50%; transform: translate(-50%, -110%); color: black; background: white; } .hide { display: none; }
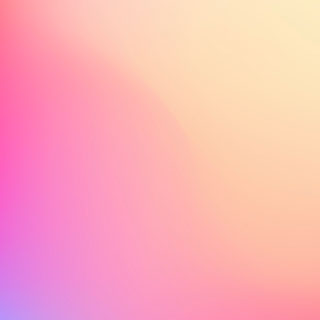
html1<!DOCTYPE html> 2<html lang="ja"> 3 4<head> 5 <meta charset="UTF-8"> 6 <meta name="viewport" content="width=device-width, initial-scale=1.0"> 7 <title>othello</title> 8 <style> 9 /* CSS 코드をここに挿入 */ 10 body { 11 text-align: center; 12 } 13 14 main { 15 display: inline-block; 16 position: relative; 17 } 18 19 /* start */ 20 #modal { 21 position: absolute; 22 top: 0; 23 left: 0; 24 width: 100%; 25 height: 100%; 26 background: rgba(31, 19, 19, 0.8); 27 color: white; 28 } 29 30 #menu { 31 display: flex; 32 justify-content: space-evenly; 33 margin-top: 220px; 34 } 35 36 h3, 37 p { 38 color: white; 39 } 40 41 #menu p { 42 width: 100px; 43 font-size: 24px; 44 padding: 3px; 45 margin: 15px; 46 border: solid 1px white; 47 cursor: pointer; 48 background: black; 49 color: white; 50 } 51 52 /* game */ 53 #board { 54 margin: 0 auto; 55 background: #555555; 56 } 57 58 td.cell { 59 background: rgb(128, 216, 154); 60 width: 46px; 61 height: 46px; 62 margin: 2px; 63 } 64 65 .cell div { 66 width: 35px; 67 height: 35px; 68 border-radius: 50%; 69 margin: 0 auto; 70 } 71 72 .cell-6>tr>td { 73 width: 63px; 74 height: 63px; 75 } 76 77 .cell-6>tr>td>div { 78 width: 50px; 79 height: 50px; 80 } 81 82 .cell-4>tr>td { 83 width: 92px; 84 height: 92px; 85 } 86 87 .cell-4>tr>td>div { 88 width: 70px; 89 height: 70px; 90 } 91 92 .black { 93 background: black; 94 } 95 96 .white { 97 background: white; 98 } 99 100 h2 { 101 text-align: center; 102 margin: 5px 0; 103 } 104 105 #counter { 106 margin: 0 auto; 107 width: 350px; 108 display: flex; 109 line-height: 50px; 110 font-weight: bold; 111 justify-content: space-between; 112 } 113 114 #numBlack { 115 font-size: 36px; 116 margin: 5px; 117 width: 100px; 118 height: 50px; 119 background: black; 120 color: white; 121 border-radius: 5px; 122 } 123 124 #numWhite { 125 font-size: 36px; 126 margin: 5px; 127 width: 100px; 128 height: 50px; 129 border: solid 1px black; 130 border-radius: 5px; 131 } 132 133 /* end */ 134 #restartBtn { 135 z-index: 1; 136 opacity: 0; 137 position: absolute; 138 width: 100%; 139 height: 50px; 140 line-height: 50px; 141 font-weight: bold; 142 cursor: pointer; 143 top: 50%; 144 left: 50%; 145 transform: translate(-50%, -110%); 146 color: black; 147 background: white; 148 } 149 150 .hide { 151 display: none; 152 } 153 </style> 154</head> 155 156<body> 157 <main> 158 <div id="modal"> 159 <h3>オセロ</h2> 160 <p>マスの数を選択してください</p> 161 <div id="menu"> 162 <p id="4" class="select">4x4</p> 163 <p id="6" class="select">6x6</p> 164 <p id="8" class="select">8x8</p> 165 </div> 166 </div> 167 <div id="restartBtn" class="hide">クリックするとスタート画面に戻ります</div> 168 <div id="content"> 169 170 <table id="board"></table> 171 </div> 172 <div id="counter" class="hide"> 173 <div id="numBlack"></div> 174 <h2></h2> 175 <div id="numWhite"></div> 176 </div> 177 </main> 178 179 <script> 180 /* JavaScript コードをここに挿入 */ 181 "use strict"; 182 183 const BLACK = 1, 184 WHITE = -1; 185 let data = []; 186 let turn = true; 187 const board = document.getElementById("board"); 188 const h2 = document.querySelector("h2"); 189 const counter = document.getElementById("counter"); 190 const modal = document.getElementById("modal"); 191 document.querySelectorAll(".select").forEach((value) => { 192 value.addEventListener("click", start); 193 }); 194 let cells = 8; // マスの数 195 196 // スタート画面でマスの数が選択された時の処理 197 function start(e) { 198 cells = Number(e.target.id); 199 board.innerHTML = ""; 200 init(); 201 modal.classList.add("hide"); 202 } 203 204 // 初期化 205 function init() { 206 for (let i = 0; i < cells; i++) { 207 const tr = document.createElement("tr"); 208 data[i] = Array(cells).fill(0); 209 for (let j = 0; j < cells; j++) { 210 const td = document.createElement("td"); 211 const disk = document.createElement("div"); 212 tr.appendChild(td); 213 td.appendChild(disk); 214 td.className = "cell"; 215 td.onclick = clicked; 216 } 217 board.appendChild(tr); 218 } 219 // マスの数によって石の初期配置を変える 220 switch (cells) { 221 case 4: 222 board.classList.add("cell-4"); 223 putDisc(1, 1, WHITE); 224 putDisc(2, 2, WHITE); 225 putDisc(1, 2, BLACK); 226 putDisc(2, 1, BLACK); 227 break; 228 case 6: 229 board.classList.add("cell-6"); 230 putDisc(2, 2, WHITE); 231 putDisc(3, 3, WHITE); 232 putDisc(2, 3, BLACK); 233 putDisc(3, 2, BLACK); 234 break; 235 case 8: 236 putDisc(3, 3, WHITE); 237 putDisc(4, 4, WHITE); 238 putDisc(3, 4, BLACK); 239 putDisc(4, 3, BLACK); 240 } 241 showTurn(); 242 } 243 244 init(); 245 246 // 石を描画 247 function putDisc(x, y, color) { 248 board.rows[y].cells[x].firstChild.className = 249 color === BLACK ? "black" : "white"; 250 board.rows[y].cells[x].animate( 251 { opacity: [0.4, 1] }, 252 { duration: 700, fill: "forwards" } 253 ); 254 data[y][x] = color; 255 } 256 257 // 手番などの表示 258 function showTurn() { 259 h2.textContent = turn ? "黒の番です" : "白の番です"; 260 let numWhite = 0, 261 numBlack = 0, 262 numEmpty = 0; 263 for (let x = 0; x < cells; x++) { 264 for (let y = 0; y < cells; y++) { 265 if (data[x][y] === WHITE) { 266 numWhite++; 267 } 268 if (data[x][y] === BLACK) { 269 numBlack++; 270 } 271 if (data[x][y] === 0) { 272 numEmpty++; 273 } 274 } 275 } 276 document.getElementById("numBlack").textContent = numBlack; 277 document.getElementById("numWhite").textContent = numWhite; 278 279 let blacDisk = checkReverse(BLACK); 280 let whiteDisk = checkReverse(WHITE); 281 282 if (numWhite + numBlack === cells * cells || (!blacDisk && !whiteDisk)) { 283 if (numBlack > numWhite) { 284 document.getElementById("numBlack").textContent = numBlack + numEmpty; 285 h2.textContent = "黒の勝ち!!"; 286 restartBtn(); 287 showAnime(); 288 } else if (numBlack < numWhite) { 289 document.getElementById("numWhite").textContent = numWhite + numEmpty; 290 h2.textContent = "白の勝ち!!"; 291 restartBtn(); 292 showAnime(); 293 } else { 294 h2.textContent = "引き分け"; 295 restartBtn(); 296 showAnime(); 297 } 298 return; 299 } 300 if (!blacDisk && turn) { 301 h2.textContent = "黒スキップ"; 302 showAnime(); 303 turn = !turn; 304 setTimeout(showTurn, 2000); 305 return; 306 } 307 if (!whiteDisk && !turn) { 308 h2.textContent = "白スキップ"; 309 showAnime(); 310 turn = !turn; 311 setTimeout(showTurn, 2000); 312 return; 313 } 314 } 315 316 // マスがクリックされた時の処理 317 function clicked() { 318 const color = turn ? BLACK : WHITE; 319 const y = this.parentNode.rowIndex; 320 const x = this.cellIndex; 321 // マスに置けるかチェック 322 if (data[y][x] !== 0) { 323 return; 324 } 325 const result = checkPut(x, y, color); 326 if (result.length > 0) { 327 result.forEach((value) => { 328 putDisc(value[0], value[1], color); 329 }); 330 turn = !turn; 331 } 332 showTurn(); 333 } 334 335 // 置いたマスの周囲8方向をチェック 336 function checkPut(x, y, color) { 337 let dx, dy; 338 const opponentColor = color == BLACK ? WHITE : BLACK; 339 let tmpReverseDisk = []; 340 let reverseDisk = []; 341 // 周囲8方向を調べる配列 342 const direction = [ 343 [-1, 0], // 左 344 [-1, 1], // 左下 345 [0, 1], // 下 346 [1, 1], // 右下 347 [1, 0], // 右 348 [1, -1], // 右上 349 [0, -1], // 上 350 [-1, -1], // 左上 351 ]; 352 353 // すでに置いてある 354 if (data[y][x] === BLACK || data[y][x] === WHITE) { 355 return []; 356 } 357 // 置いた石の周りに違う色の石があるかチェック 358 for (let i = 0; i < direction.length; i++) { 359 dx = direction[i][0] + x; 360 dy = direction[i][1] + y; 361 if ( 362 dx >= 0 && 363 dy >= 0 && 364 dx <= cells - 1 && 365 dy <= cells - 1 && 366 opponentColor === data[dy][dx] 367 ) { 368 tmpReverseDisk.push([x, y]); 369 tmpReverseDisk.push([dx, dy]); 370 // 裏返せるかチェック 371 while (true) { 372 dx += direction[i][0]; 373 dy += direction[i][1]; 374 if ( 375 dx < 0 || 376 dy < 0 || 377 dx > cells - 1 || 378 dy > cells - 1 || 379 data[dy][dx] === 0 380 ) { 381 tmpReverseDisk = []; 382 break; 383 } 384 if (opponentColor === data[dy][dx]) { 385 tmpReverseDisk.push([dx, dy]); 386 } 387 if (color === data[dy][dx]) { 388 reverseDisk = reverseDisk.concat(tmpReverseDisk); 389 tmpReverseDisk = []; 390 break; 391 } 392 } 393 } 394 } 395 return reverseDisk; 396 } 397 398 // 裏返せる場所があるか確認 399 function checkReverse(color) { 400 for (let x = 0; x < cells; x++) { 401 for (let y = 0; y < cells; y++) { 402 const result = checkPut(x, y, color); 403 if (result.length > 0) { 404 return true; 405 } 406 } 407 } 408 return false; 409 } 410 411 // ゲーム終了画面 412 function restartBtn() { 413 const restartBtn = document.getElementById("restartBtn"); 414 restartBtn.classList.remove("hide"); 415 restartBtn.animate( 416 { opacity: [1, 0.5, 1] }, 417 { delay: 2000, duration: 3000, iterations: "Infinity" } 418 ); 419 420 restartBtn.addEventListener("click", () => { 421 document.location.reload(); 422 }); 423 } 424 425 function showAnime() { 426 h2.animate({ opacity: [0, 1] }, { duration: 500, iterations: 4 }); 427 } 428 </script> 429</body> 430 431</html>
コメント 0
他のプロンプトもチェック
GPTs
システム開発
オリジナルサインの作成のGPTs ちょっとキャラクターを乗っけています。 生成系AIは、今のところ漢字を描くのが苦手なので、ローマ字で登録ください。 (ローマ字もたまに間違えますが)211.24K2.59KGPTs
資料作成
シンプルな質問に答えるだけで、ワークフローを可視化してくれる最高の相棒 ※マーメイド記法で出力し、GPTのガイド通りマーメイドライブエディタに貼り付けるだけでフローを可視化できます。 ※業務引継ぎや、業務可視化、業務改善のお供にどうぞ!10182215画像生成
キャラクター作成
推奨モデル - Midjourneyゴッホ風のイラストを生成します。追加して欲しい選択肢がありましたらコメントください♪ #midjourney #アイコン #Instagram #X #Twitter #SNS #画像生成10945